Viam Documentation
Viam integrates with hardware and software on any device in the physical world. Once you set up your machines, you can use Viam SDKs to program your devices and connected hardware. Everything is managed in the cloud and you can add use machine learning, data management, and much more for your projects.
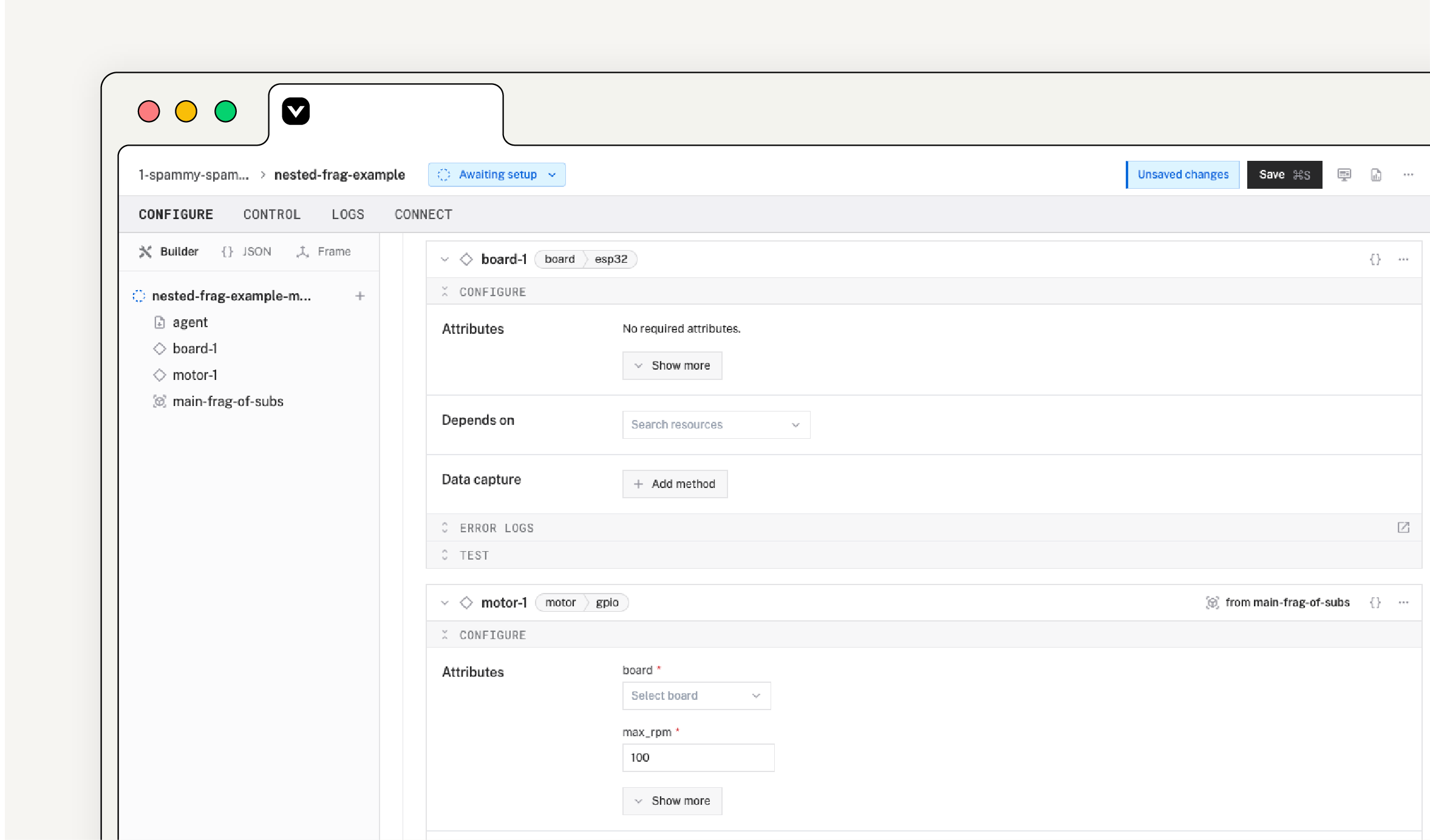
The Viam platform
Viam allows you to control and program any sensor, actuator or other hardware that is connected to a device. The Viam platform offers builtin capabilities to capture data from devices to the cloud, to build and deploy machine learning models, to alert on problems, and much more. With the connection to the cloud, you can configure, control, and manage your devices from anywhere.
Build & integrate
To get started, install Viam on any device and integrate your hardware. Then you can control your device and any attached physical hardware securely from anywhere in the world.
Work with Data and AI
Viam's data and AI capabilities enable you to capture and sync or upload data, build a dataset, train and deploy ML models, and run inference with computer vision. Then, you can act or alert on inferences.
Deploy, manage, and troubleshoot
Viam’s fleet management tooling allows you to remotely deploy and manage software on any fleet of devices. You can monitor all connected devices and troubleshoot any issues - from anywhere.