Run SDK Code
After saving your code sample and adding control logic with Viam’s SDKs, run your program to control your Viam-connected machine.
Authentication
To authenticate yourself to your machine, you need
The machine part’s API key:
To authenticate, use a machine part API key or an API key with access to the machine. Copy and paste the API key ID and the API key into your environment variables or directly into the code:
async def connect(): opts = RobotClient.Options.with_api_key( # Replace "<API-KEY>" (including brackets) with your machine's API key api_key='<API-KEY>', # Replace "<API-KEY-ID>" (including brackets) with your machine's API key # ID api_key_id='<API-KEY-ID>' ) return await RobotClient.at_address('ADDRESS FROM THE VIAM APP', opts)
robot, err := client.New( context.Background(), "ADDRESS FROM THE VIAM APP", logger, client.WithDialOptions(rpc.WithEntityCredentials( // Replace "<API-KEY-ID>" (including brackets) with your machine's API key ID "<API-KEY-ID>", rpc.Credentials{ Type: rpc.CredentialsTypeAPIKey, // Replace "<API-KEY>" (including brackets) with your machine's API key Payload: "<API-KEY>", })), )
// Replace with the host of your actual machine running Viam. const host = "ADDRESS FROM THE VIAM APP"; const robot = await VIAM.createRobotClient({ host, credential: { type: "api-key", // Replace "<API-KEY>" (including brackets) with your machine's API key payload: "<API-KEY>", }, // Replace "<API-KEY-ID>" (including brackets) with your machine's API key ID authEntity: "<API-KEY-ID>", signalingAddress: "https://app.viam.com:443", });
std::string host("ADDRESS FROM THE VIAM APP"); DialOptions dial_opts; dial_opts.set_type("api-key"); // Replace "<API-KEY-ID>" with your machine's API key ID dial_opts.set_entity("<API-KEY-ID>"); // Replace "<API-KEY>" with your machine's API key Credentials credentials("<API-KEY>"); dial_opts.set_credentials(credentials); boost::optional<DialOptions> opts(dial_opts); Options options(0, opts); auto robot = RobotClient::at_address(host, options);
Future<void> connectToViam() async { const host = 'ADDRESS FROM THE VIAM APP'; // Replace '<API-KEY-ID>' (including brackets) with your API key ID const apiKeyID = '<API-KEY-ID>'; // Replace '<API-KEY>' (including brackets) with your API key const apiKey = '<API-KEY>'; final robot = await RobotClient.atAddress( host, RobotClientOptions.withApiKey(apiKeyId, apiKey), ); print(robot.resourceNames); }
Caution
Do not share your machine part API key or machine address publicly. Sharing this information could compromise your system security by allowing unauthorized access to your machine, or to the computer running your machine.
Location secret (deprecated)
Prior to API keys, Viam used location secrets for authentication. Location secrets are now deprecated. To avoid connection issues, start using API keys.
The machine’s remote address:
Include the address, which resembles
12345.somemachine-main.viam.cloud
. The machine address is a public address to connect to your machine. You can find this address at the top of the machine’s CONTROL tab or on the CONNECT tab’s Code sample page.
Run code remotely
You can remotely control your machine from anywhere in the world. If your machine and your personal computer are both connected to the Internet, you can run code to control your machine remotely from your personal computer.
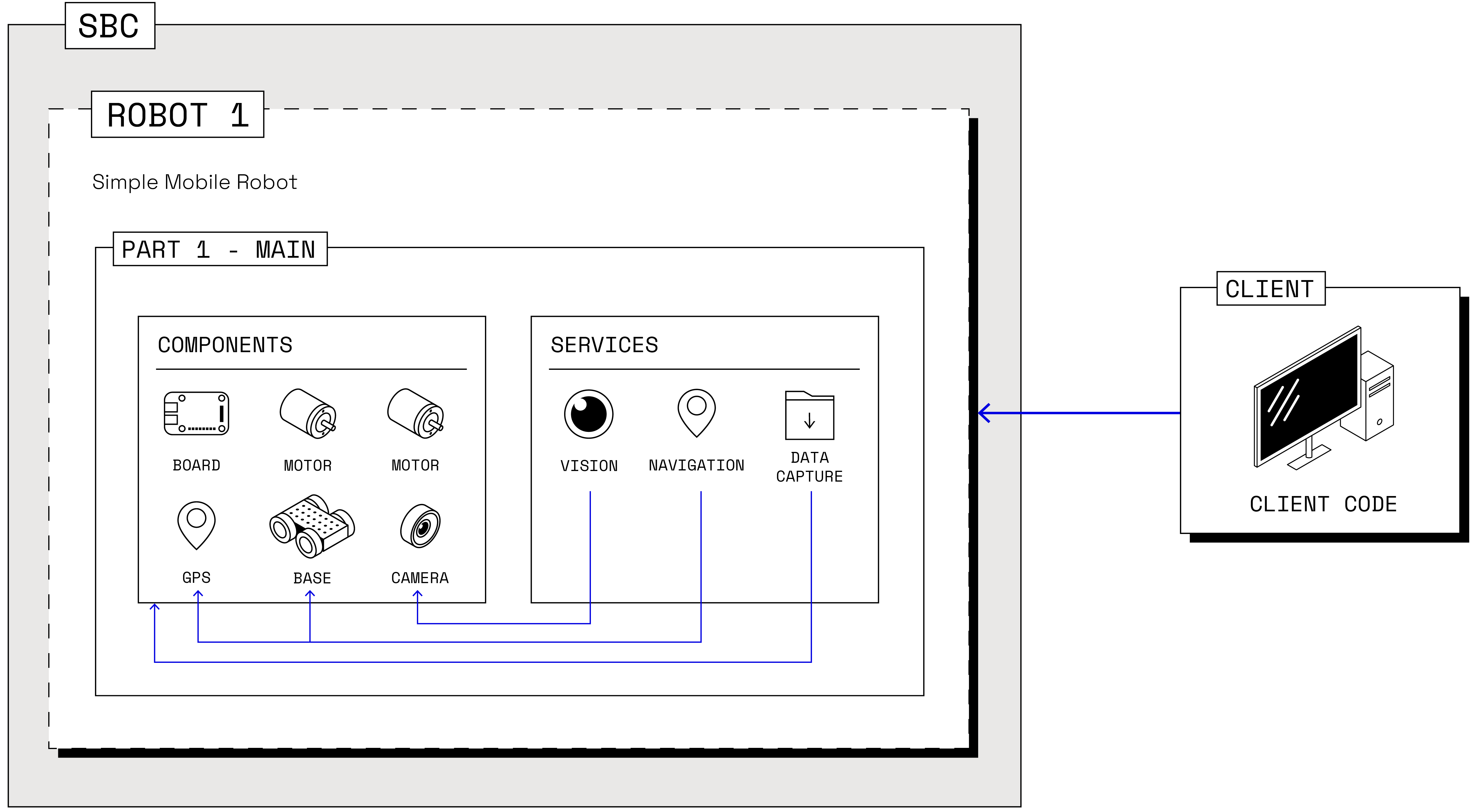
This method is convenient for most use cases because your machine and your personal computer do not have to be connected to the same WAN/LAN to issue control commands.
When you run code on one computer, creating a client session, the code running on that computer sends instructions to your machine’s viam-server
instance over the Internet.
After editing your code to include your machine’s authentication credentials, run a command to execute the program in the terminal of a computer with the appropriate programming language and Viam SDK installed:
python3 ~/myCode/myViamFile.py
go run ~/myCode/myViamFile.go
For an example, see this execution demo.
For information on running C++ code see the instructions on GitHub.
flutter run <DART_FILE>
As long as that computer is able to establish a network connection with the machine’s computer, your control logic will be executed on the machine.
If the internet becomes unavailable to the machine or to your computer but a local network is available, your code will continue to run as described in the next section:
Run code on local network
Your machines do not need to be connected to the Internet for you to be able to run code. As long as your machine is connected to the same LAN or WAN network as the device running the code, you can connect to it and run code.
When you use the connection code sample from the CONNECT tab, that code establishes a client session that automatically uses the most efficient route to send commands to your machine. That means that when the device your code runs on is on the same network as your machine, even if internet is available, the connection will choose the most efficient route and connect over LAN or WAN. If you subsequently lose internet connectivity, but stay connected to LAN or WAN, the connection will thus remain.
Run code on-machine
You can run SDK code directly on your machine.
If you run PID control loops or your machines have intermittent or no network connectivity, you can ensure lags in communication do not interfere with the machine’s performance by running the control code on the same board that is running viam-server
.
Running everything on one machine is also convenient if you have a machine (for example, an air quality sensor) that runs all the time, and you don’t want to have to connect to it from a separate computer constantly.
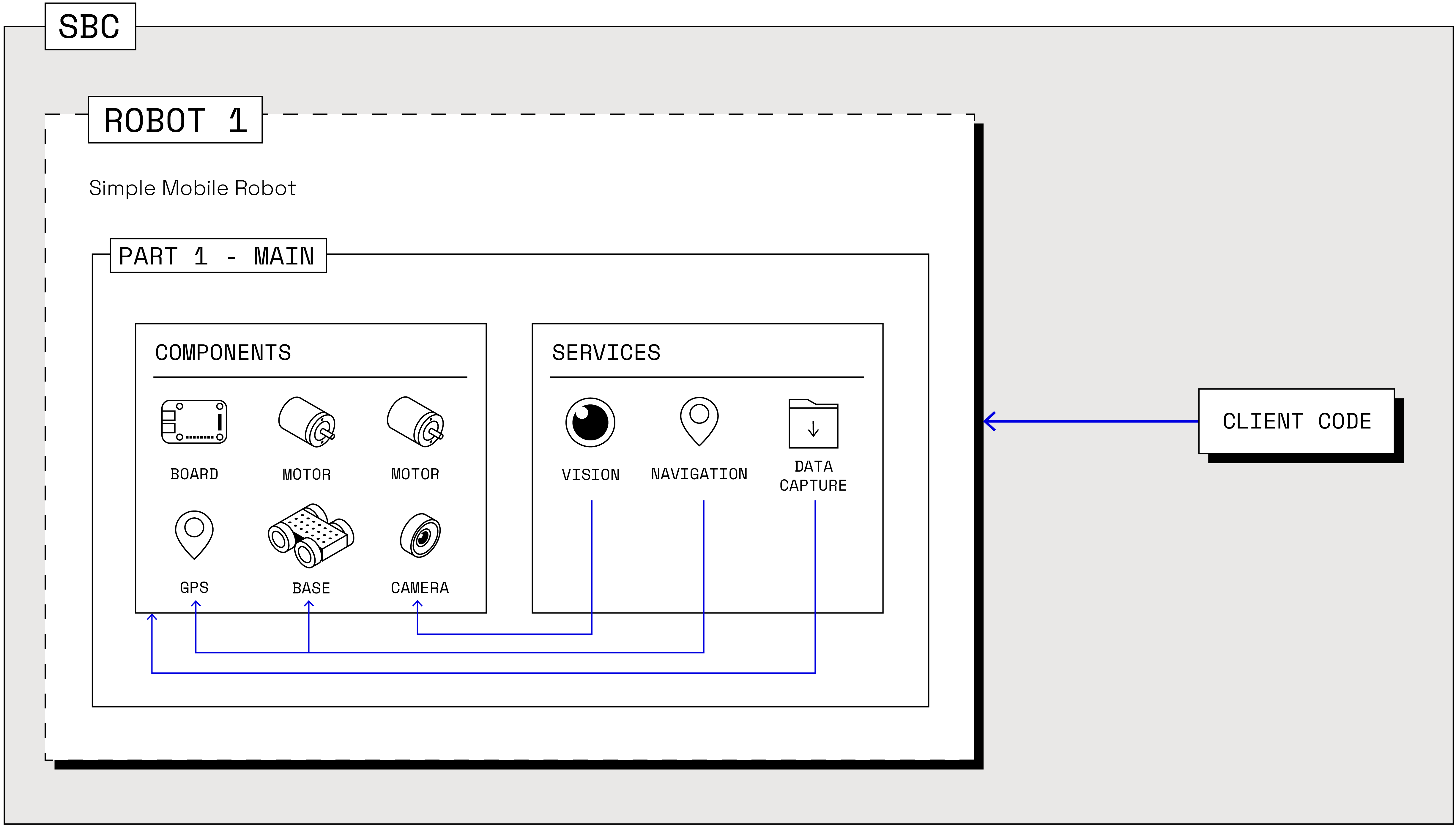
The script you run on-machine is the same as the script you run remotely or on a local network.
When the connection code from the CONNECT tab’s Code sample page executes, it creates a client session connected to your machine using the most efficient route.
Because the code is running on the same machine as viam-server
, the favored route for commands is automatically over localhost.
Install the appropriate programming language and Viam SDK on your machine and run a command to execute the program in the terminal of that machine instead of from a separate computer:
python3 ~/myCode/myViamFile.py
go run ~/myCode/myViamFile.go
For an example, see this execution demo.
For information on running C++ code see the instructions on GitHub.
flutter run <DART_FILE>
Run code automatically as a process
If you want to run your code on-machine automatically when your machine boots, you can configure your machine to run your code as a process. You can configure the process to run once on boot, or continuously.
Find information on how to configure a process in Processes.
Have questions, or want to meet other people working on robots? Join our Community Discord.
If you notice any issues with the documentation, feel free to file an issue or edit this file.
Was this page helpful?
Glad to hear it! If you have any other feedback please let us know:
We're sorry about that. To help us improve, please tell us what we can do better:
Thank you!