Board Component
A board component on your machine communicates with the other components of the machine.
A board can be:
- A single-board computer (SBC) with GPIO pins and a CPU capable of running
viam-server
. - A GPIO peripheral device that must connect to an external computer.
- A PWM peripheral device that must connect to an SBC that has a CPU and GPIO pins.
The board of a machine is also its signal wire hub that provides access to general purpose input/output (GPIO) pins: a collection of pins on the motherboard of a computer that can receive electrical signals.
Signaling is overseen by a computer running viam-server
which allows you to control the flow of electricity to these pins to change their state between “high” (active) and “low” (inactive), and wire them to send digital signals to and from other hardware.
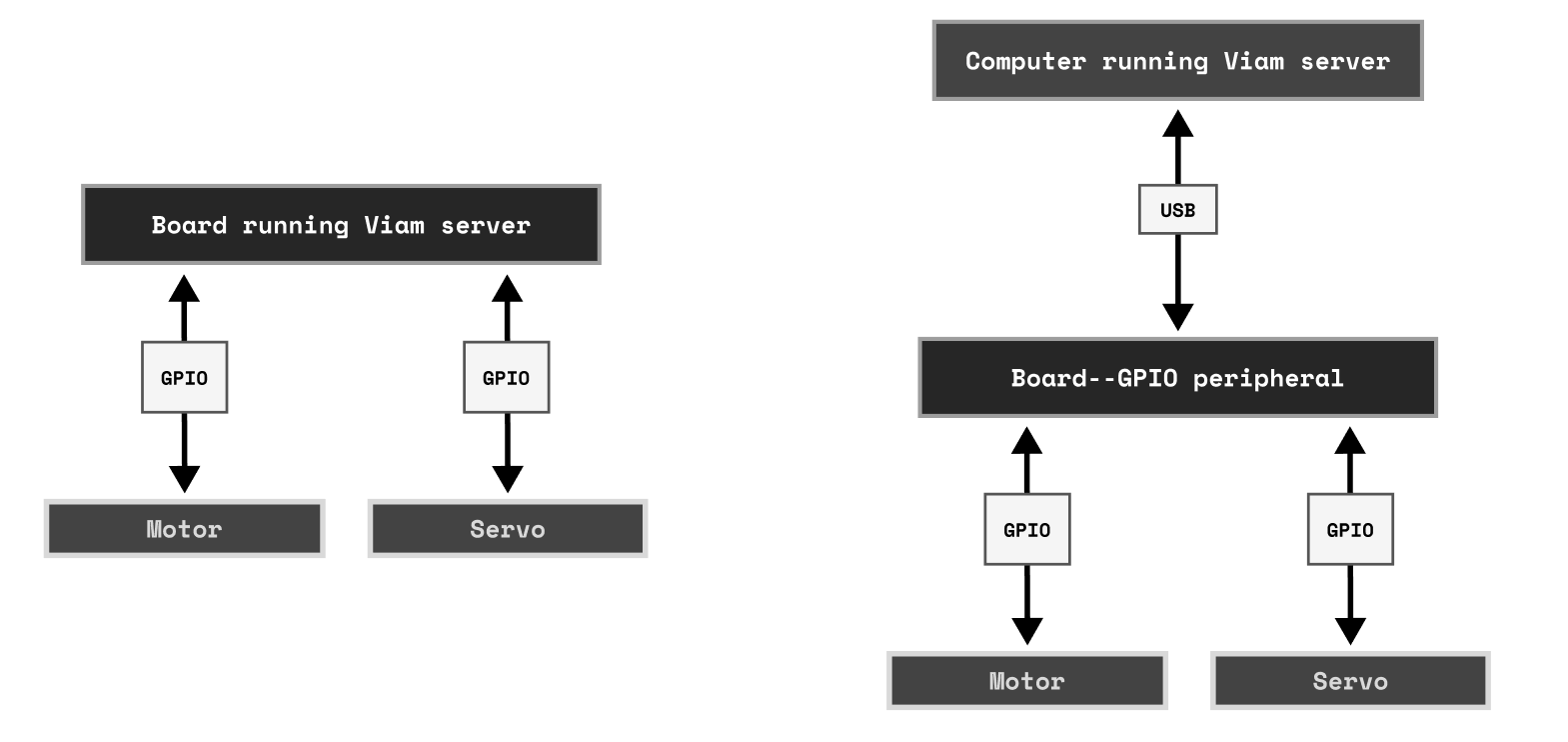
Supported models
To use your board with Viam, check whether one of the following built-in models or modular resources supports your board.
Running viam-server
The board component allows you to use the pins on your board. If there is no board model for your board:
- you can still run
viam-server
if your board supports it - you can still access USB ports
Built-in models
For configuration information, click on the model name:
Model | Description |
---|---|
pi | Raspberry Pi 4, Raspberry Pi 3 or Raspberry Pi Zero 2 W |
jetson | NVIDIA Jetson AGX Orin, NVIDIA Jetson Orin Nano, NVIDIA Jetson Xavier NX, NVIDIA Jetson Nano |
upboard | An Intel-based board like the UP4000 |
ti | Texas Instruments TDA4VM |
beaglebone | BeagleBoard’s BeagleBone AI-64 |
numato | Numato GPIO Modules, peripherals for adding GPIO pins |
pca9685 | PCA9685 Arduino I2C Interface, a 16-channel I2C PWM/servo driver peripheral |
customlinux | A model for other Linux boards. |
fake | A model used for testing, with no physical hardware |
Modular resources
Search for additional board models that you can add from the Viam Registry:
For configuration information, click on the model name:
Add support for other models
If none of the existing models fit your use case, you can create a modular resource to add support for it.
Micro-RDK
If you are using the micro-RDK, navigate to Micro-RDK Board for supported model information.
Attribute configuration
Configuring these attributes on your board allows you to integrate analog-to-digital converters and digital interrupts into your machine.
analogs
An analog-to-digital converter (ADC) takes a continuous voltage input (analog signal) and converts it to an discrete integer output (digital signal).
ADCs are useful when building a robot, as they enable your board to read the analog signal output by most types of sensors and other hardware components.
To integrate an ADC into your machine, you must first physically connect the pins on your ADC to your board.
Then, integrate analogs
into the attributes
of your board by following the Config Builder instructions or by adding the following to your board’s JSON configuration:
On your board’s panel, click Show more, then select Add analog. Assign a name to your analog and then fill in the required properties outlined below.
// "attributes": { ... ,
"analogs": [
{
"name": "<your-analog-reader-name>",
"pin": "<pin-number-on-adc>",
"spi_bus": "<your-spi-bus-index>",
"chip_select": "<chip-select-index>",
"average_over_ms": <int>,
"samples_per_sec": <int>
}
]
{
"components": [
{
"model": "pi",
"name": "your-board",
"type": "board",
"attributes": {
"analogs": [
{
"name": "current",
"pin": "1",
"spi_bus": "1",
"chip_select": "0"
},
{
"name": "pressure",
"pin": "0",
"spi_bus": "1",
"chip_select": "0"
}
]
}
}
]
}
The following properties are available for analogs
:
Name | Type | Inclusion | Description |
---|---|---|---|
name | string | Required | Your name for the analog reader. |
pin | string | Required | The pin number of the ADC’s connection pin, wired to the board. This should be labeled as the physical index of the pin on the ADC. |
chip_select | string | Required | The chip select index of the board’s connection pin, wired to the ADC. |
spi_bus | string | Required | The index of the SPI bus connecting the ADC and board. |
average_over_ms | int | Optional | Duration in milliseconds over which the rolling average of the analog input should be taken. |
samples_per_sec | int | Optional | Sampling rate of the analog input in samples per second. |
Test analogs
Once you have configured your analogs, navigate to the CONTROL tab to monitor analog values. The numbers displayed next to each analog name represent the digital signal received from the analog inputs.
digital_interrupts
Interrupts are a method of signaling precise state changes. Configuring digital interrupts to monitor GPIO pins on your board is useful when your application needs to know precisely when there is a change in GPIO value between high and low.
- When an interrupt configured on your board processes a change in the state of the GPIO pin it is configured to monitor, it calls
Tick()
to record the state change and notify any interested callbacks to “interrupt” the program. - Calling
GetGPIO()
on a GPIO pin, which you can do without configuring interrupts, is useful when you want to know a pin’s value at specific points in your program, but is less precise and convenient than using an interrupt.
Integrate digital_interrupts
into your machine in the attributes
of your board by following the Config Builder instructions, or by adding the following to your board’s JSON configuration:
On your board’s panel, click Show more, then select Add digital interrupt. Assign a name to your digital interrupt and then enter a pin number.
// "attributes": { ... ,
"digital_interrupts": [
{
"name": "<your-digital-interrupt-name>",
"pin": "<pin-number>"
}
]
{
"components": [
{
"model": "pi",
"name": "your-board",
"type": "board",
"attributes": {
"digital_interrupts": [
{
"name": "your-interrupt-1",
"pin": "15"
},
{
"name": "your-interrupt-2",
"pin": "16"
}
]
}
}
]
}
The following properties are available for digital_interrupts
:
Name | Type | Inclusion | Description |
---|---|---|---|
name | string | Required | Your name for the digital interrupt. |
pin | string | Required | The pin number of the board’s GPIO pin that you wish to configure the digital interrupt for. |
type | string | Optional | Only applies to pi model boards.
|
Test digital interrupts
Once you have configured your digital interrupts, navigate to the CONTROL tab to monitor interrupt activity. The value displayed next to each interrupt name represent the total count of interrupts triggered by the corresponding digital interrupt.
Control your board with Viam’s client SDK libraries
To get started using Viam’s SDKs to connect to and control your machine, go to your machine’s page on the Viam app, navigate to the CONNECT tab’s Code sample page, select your preferred programming language, and copy the sample code generated.
API key and API key ID
By default, the sample code does not include your machine API key and API key ID. We strongly recommend that you add your API key and API key ID as an environment variable and import this variable into your development environment as needed.
To show your machine’s API key and API key ID in the sample code, toggle Include secret on the CONNECT tab’s Code sample page.
Caution
Do not share your API key or machine address publicly. Sharing this information could compromise your system security by allowing unauthorized access to your machine, or to the computer running your machine.
When executed, this sample code will create a connection to your machine as a client.
Then control your machine programmatically by getting your board
component from the machine with FromRobot
and adding API method calls, as shown in the following examples.
These examples assume you have a board called “my_board” configured as a component of your machine.
If your board has a different name, change the name
in the code.
Be sure to import the board package for the SDK you are using:
from viam.components.board import Board
import (
"go.viam.com/rdk/components/board"
)
API
The board component supports the following methods:
Method Name | Description |
---|---|
ReadAnalog | Get an Analog by name . |
GetDigitalInterruptValue | Get a DigitalInterrupt by name . |
GetGPIO | Get a GPIOPin by its pin number. |
SetGPIO | Set a GPIOPin by its pin number. |
AnalogNames | Get the name of every Analog . |
DigitalInterruptNames | Get the name of every DigitalInterrupt . |
Status | Get the current status of this board. |
SetPWM | Set the board to the indicated power mode. |
WriteAnalog | Write an analog value to a pin on the board. |
StreamTicks | Start a stream of DigitalInterrupt ticks. |
GetGeometries | Get all the geometries associated with the board in its current configuration, in the frame of the board. |
DoCommand | Send or receive model-specific commands. |
Close | Safely shut down the resource and prevent further use. |
Additionally, the nested GPIOPin
, Analog
, and DigitalInterrupt
interfaces support the following methods:
GPIOPin
API:
Method Name | Description |
---|---|
SetGPIO | Set the output of this pin to high/low. |
GetGPIO | Get if this pin is active (high). |
GetPWM | Get the pin’s pulse-width modulation duty cycle. |
SetPWM | Set the pin’s pulse-width modulation duty cycle. |
PWMFreq | Get the pulse-width modulation frequency of this pin. |
SetPWMFreq | Set the pulse-width modulation frequency of this pin. |
Close | Safely shut down the resource and prevent further use. |
Analog
API:
Method Name | Description |
---|---|
Read | Read the current integer value of the digital signal output by the ADC. |
Close | Safely shut down the resource and prevent further use. |
DigitalInterrupt
API:
Method Name | Description |
---|---|
Value | Get the current value of this interrupt. |
Tick | Record an interrupt. |
AddCallback | Add a channel as a callback for Tick(). |
Close | Safely shut down the resource and prevent further use. |
ReadAnalog
Get an Analog
pin by name
.
Parameters:
name
(str): Name of the analog pin you want to retrieve.
Returns:
- (Analog): An interface representing an analog pin configured and residing on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the Analog "my_example_analog_pin".
analog = await my_board.analog_by_name(name="my_example_analog")
Parameters:
name
(string): Name of the analog pin you want to retrieve. Set as the"name"
property in configuration.
Returns:
- (Analog): An interface representing an analog pin configured and residing on the board.
- (bool): True if there was an analog pin of this
name
found on your board.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the Analog pin "my_example_analog".
analog, err := myBoard.AnalogByName("my_example_analog")
// Read the value from the analog pin.
val, err := analog.Read(context.Background, map[string]interface{})
GetDigitalInterruptValue
Get an DigitalInterrupt
by name
.
Parameters:
name
(str): Name of the digital interrupt you want to retrieve. Set as the"name"
property in configuration.
Returns:
- (DigitalInterrupt): An interface representing a configured interrupt on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt = await my_board.digital_interrupt_by_name(
name="my_example_digital_interrupt")
Parameters:
name
(string): Name of the digital interrupt you want to retrieve. Set as the"name"
property in configuration.
Returns:
- (DigitalInterrupt): An interface representing a configured interrupt on the board.
- (bool): True if there was a digital interrupt of this
name
found on your board.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
GPIOPinByName
Get a GPIOPin
by pin number.
Parameters:
name
(str): Pin number of the GPIO pin you want to retrieve as aGPIOPin
interface. Refer to the pinout diagram and data sheet of your board model for pin numbers.
Returns:
- (GPIOPin): An interface representing an individual GPIO pin on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
Parameters:
name
(string): pin number of the GPIO pin you want to retrieve as aGPIOPin
interface. Refer to the pinout diagram and data sheet of your board model for pin numbers.
Returns:
- (GPIOPin): An interface representing an individual GPIO pin on the board.
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
AnalogNames
Get the name of every Analog
pin configured and residing on the board or implemented in the board driver.
Parameters:
- None
Returns:
- (List[str]): A list containing the
"name"
of every analog pin configured on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the name of every Analog configured on the board.
names = await my_board.analog_names()
Parameters:
- None
Returns:
- ([]string): A slice containing the
"name"
of every analog pin configured on the board.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the name of every Analog pin configured on the board.
names := myBoard.AnalogNames()
DigitalInterruptNames
Get the name of every DigitalInterrupt
configured on the board.
Parameters:
- None
Returns:
- (List[str]): A list containing the
"name"
of every interrupt configured on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the name of every DigitalInterrupt configured on the board.
names = await my_board.digital_interrupt_names()
Parameters:
- None
Returns:
- ([]string): A slice containing the
"name"
of every interrupt configured on the board.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the name of every DigitalInterrupt configured on the board.
names := myBoard.DigitalInterruptNames()
Status
Get the current status of the board as a BoardStatus
.
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (BoardStatus): Mappings of the current status of the fields and values of any Analog pins and DigitalInterrupts configured on the board.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the current status of the board.
status = await my_board.status()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
- (BoardStatus): Mappings of the current status of the fields and values of any AnalogReaders and DigitalInterrupts configured on the board.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the current status of the board.
err := myBoard.Status(context.Background(), nil)
SetPowerMode
Set the board to the indicated PowerMode
.
Info
This method may not receive a successful response from gRPC when you set the board to the offline power mode PowerMode.POWER_MODE_OFFLINE_DEEP
.
When this is the case for your board model, the call is returned with an error specifying that the remote procedure call timed out or that the endpoint is no longer available. This is expected: the board has been successfully powered down and can no longer respond to messages.
Parameters:
mode
(PowerMode): Options to specify power usage of the board:PowerMode.POWER_MODE_UNSPECIFIED
,PowerMode.POWER_MODE_NORMAL
, andPowerMode.POWER_MODE_OFFLINE_DEEP
.duration
(Optional[datetime.timedelta]): If provided, the board will exit the given power mode after the specified duration.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- None
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Set the power mode of the board to OFFLINE_DEEP.
status = await my_board.set_power_mode(mode=PowerMode.POWER_MODE_OFFLINE_DEEP)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.mode
(PowerMode): Options to specify power usage of the board:boardpb.PowerMode_POWER_MODE_UNSPECIFIED
,boardpb.PowerMode_POWER_MODE_NORMAL
, andboardpb.PowerMode_POWER_MODE_OFFLINE_DEEP
.duration
(*time.Duration): If provided, the board will exit the given power mode after the specified duration.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Set the power mode of the board to OFFLINE_DEEP.
err := myBoard.Status(context.Background(), nil)
myBoard.SetPowerMode(context.Background(), boardpb.PowerMode_POWER_MODE_OFFLINE_DEEP, nil)
WriteAnalog
Write an analog value to a pin on the board.
Parameters:
pin
(string): Name of the pin (pin number).value
(int): Value to write to the pin.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- None
my_board = Board.from_robot(robot=robot, name="my_board")
# Set pin 11 to value 48.
await my_board.write_analog(pin="11", value=48)
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.pin
(string): Name of the pin (pin number).value
(int): Value to write to the pin.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Set pin 11 to value 48.
err := myBoard.WriteAnalog(context.Background(), "11", 48, nil)
StreamTicks
Start a stream of DigitalInterrupt
ticks.
Parameters:
interrupts
(List[DigitalInterrupt]): List of digital interrupts to receive ticks from.
Returns:
- (
TickStream
): Stream of Ticks, objects containingpin_name
,time
, andhigh
fields.
my_board = Board.from_robot(robot=robot, name="my_board")
di8 = await my_board.digital_interrupt_by_name(name="8")
di11 = await my_board.digital_interrupt_by_name(name="11")
# Stream ticks from the listed digital interrupts on pins 8 and 11.
ticks = await my_board.stream_ticks([di8, di11])
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.interrupts
([]DigitalInterrupt): Slice of digital interrupts to receive ticks from.ch
(chan Tick): The channel to stream Ticks, structs containingName
,High
, andTimestampNanosec
fields.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Make a channel to stream ticks
ticksChan := make(chan board.Tick)
interrupts := []*DigitalInterrupt{}
if di8, err := myBoard.DigitalInterruptByName("8"); err == nil {
interrupts = append(interrupts, di8)
}
if di11, err := myBoard.DigitalInterruptByName("11"); err == nil {
interrupts = append(interrupts, di11)
}
// Stream ticks on ticksChan from the listed digital interrupts on pins 8 and 11.
err = myBoard.StreamTicks(context.Background(), interrupts, ticksChan, nil)
GetGeometries
Get all the geometries associated with the board in its current configuration, in the frame of the board. The motion and navigation services use the relative position of inherent geometries to configured geometries representing obstacles for collision detection and obstacle avoidance while motion planning.
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (List[Geometry]): The geometries associated with the board, in any order.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
geometries = await my_board.get_geometries()
if geometries:
# Get the center of the first geometry
print(f"Pose of the first geometry's centerpoint: {geometries[0].center}")
DoCommand
Execute model-specific commands that are not otherwise defined by the component API.
For built-in models, model-specific commands are covered with each model’s documentation.
If you are implementing your own board and add features that have no built-in API method, you can access them with DoCommand
.
Parameters:
command
(Dict[str, Any]): The command to execute.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (Dict[str, Any]): Result of the executed command.
my_board = Board.from_robot(robot=robot, name="my_board")
my_command = {
"command": "dosomething",
"someparameter": 52
}
await my_board.do_command(my_command)
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.cmd
(map[string]interface{}): The command to execute.
Returns:
- (map[string]interface{}): Result of the executed command.
- (error): An error, if one occurred.
myBoard, err := board.FromRobot(robot, "my_board")
resp, err := myBoard.DoCommand(ctx, map[string]interface{}{"command": "dosomething", "someparameter": 52})
For more information, see the Go SDK Code.
Close
Safely shut down the resource and prevent further use.
Parameters:
- None
Returns:
- None
my_board = Board.from_robot(robot, "my_board")
await my_board.close()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error) : An error, if one occurred.
myBoard, err := board.FromRobot(robot, "my_board")
err := myBoard.Close(ctx)
For more information, see the Go SDK Docs.
GPIOPin
API
SetGPIO
Set the digital signal output of this pin to low (0V) or high (active, >0V).
Parameters:
high
(bool): Iftrue
, set the state of the pin to high. Iffalse
, set the state of the pin to low.extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- None
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Set the pin to high.
await pin.set(high="true")
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.high
(bool): Iftrue
, set the state of the pin to high. Iffalse
, set the state of the pin to low.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Set the pin to high.
err := pin.Set(context.Background(), "true", nil)
GetGPIO
Get if the digital signal output of this pin is high (active, >0V).
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (bool): If
true
, the state of the pin is high. Iffalse
, the state of the pin is low.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Get if it is true or false that the state of the pin is high.
high = await pin.get()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (bool): If
true
, the state of the pin is high. Iffalse
, the state of the pin is low. - (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Get if it is true or false that the state of the pin is high.
high := pin.Get(context.Background(), nil)
GetPWM
Info
Pulse-width modulation (PWM) is a method where of transmitting a digital signal in the form of pulses to control analog circuits. With PWM on a board, the continuous digital signal output by a GPIO pin is sampled at regular intervals and transmitted to any hardware components wired to the pin that read analog signals. This enables the board to communicate with these components.
Get the pin’s pulse-width modulation (PWM) duty cycle: a float [0.0
, 1.0
] representing the percentage of time the digital signal output by this pin is in the high state (active, >0V) relative to the interval period of the PWM signal (interval period being the mathematical inverse of the PWM frequency).
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (float): A float [
0.0
,1.0
] representing the percentage of time the digital signal output by this pin is in the high state relative to the interval period of the PWM signal.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Get if it is true or false that the state of the pin is high.
duty_cycle = await pin.get_pwm()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (float64): A float [
0.0
,1.0
] representing the percentage of time the digital signal output by this pin is in the high state relative to the interval period of the PWM signal. - (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Get if it is true or false that the state of the pin is high.
duty_cycle := pin.PWM(context.Background(), nil)
SetPWM
Set the pin’s Pulse-width modulation (PWM) duty cycle: a float [0.0
, 1.0
] indicating the percentage of time the digital signal output of this pin is in the high state (active, >0V) relative to the interval period of the PWM signal (interval period being the mathematical inverse of the PWM frequency).
Parameters:
cycle
(float64): A float [0.0
,1.0
] representing the percentage of time the digital signal output by this pin is in the high state relative to the interval period of the PWM signal.extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (bool): If
true
, the state of the pin is high. Iffalse
, the state of the pin is low.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Set the duty cycle to .6, meaning that this pin will be in the high state for
# 60% of the duration of the PWM interval period.
await pin.set_pwm(cycle=.6)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.cycle
(float64): A float [0.0
,1.0
] representing the percentage of time the digital signal output by this pin is in the high state relative to the interval period of the PWM signal.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Set the duty cycle to .6, meaning that this pin will be in the high state for 60% of the duration of the PWM interval period.
err := pin.SetPWM(context.Background(), .6, nil)
PWMFreq
Get the Pulse-width modulation (PWM) frequency in Hertz (Hz) of this pin, the count of PWM interval periods per second. The optimal value for PWM Frequency depends on the type and model of component you control with the signal output by this pin. Refer to your device’s data sheet for PWM Frequency specifications.
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (int): The PWM Frequency in Hertz (Hz) (the count of PWM interval periods per second) the digital signal output by this pin is set to.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Get the PWM frequency of this pin.
freq = await pin.get_pwm_frequency()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (unit): The PWM Frequency in Hertz (Hz) (the count of PWM interval periods per second) the digital signal output by this pin is set to.
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Get the PWM frequency of this pin.
freqHz, err := pin.PWMFreq(context.Background(), nil)
SetPWMFreq
Set the Pulse-width modulation (PWM) frequency in Hertz (Hz) of this pin, the count of PWM interval periods per second. The optimal value for PWM Frequency depends on the type and model of component you control with the PWM signal output by this pin. Refer to your device’s data sheet for PWM Frequency specifications.
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (int): The PWM Frequency in Hertz (Hz), the count of PWM interval periods per second, to set the digital signal output by this pin to.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Set the PWM frequency of this pin to 1600 Hz.
high = await pin.set_pwm_frequency(frequency=1600)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.freqHz
(unit): The PWM Frequency in Hertz (Hz), the count of PWM interval periods per second, to set the digital signal output by this pin to.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Set the PWM frequency of this pin to 1600 Hz.
high := pin.SetPWMFreq(context.Background(), 1600, nil)
Close
Safely shut down the resource and prevent further use.
Parameters:
- None
Returns:
- None
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Close the pin.
await pin.close()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error) : An error, if one occurred.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the GPIOPin with pin number 15.
pin, err := myBoard.GPIOPinByName("15")
// Close the pin.
err := pin.Close(ctx)
For more information, see the Go SDK Docs.
Analog
API
Read
Read the current integer value of the digital signal output by the ADC.
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (int): The value of the digital signal output by the analog pin.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the GPIOPin with pin number 15.
pin = await my_board.gpio_pin_by_name(name="15")
# Get if it is true or false that the pin is set to high.
duty_cycle = await pin.get_pwm()
# Get the Analog pin "my_example_analog".
analog = await my_board.analog_by_name(
name="my_example_analog")
# Get the value of the digital signal "my_example_analog" has most
# recently measured.
reading = analog.read()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the Analog pin "my_example_analog".
analog, err := myBoard.AnalogByName("my_example_analog")
// Get the value of the digital signal "my_example_analog" has most recently measured.
reading := analog.Read(context.Background(), nil)
Close
Safely shut down the resource and prevent further use.
Parameters:
- None
Returns:
- None
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the Analog pin "my_example_analog".
analog = await my_board.analog_by_name(
name="my_example_analog")
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error) : An error, if one occurred.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the Analog "my_example_analog".
analog, err := myBoard.AnalogByName("my_example_analog")
// Read the current value from the analog pin.
value, err := analog.Read(context.Background(), map[string]interface{})
For more information, see the Go SDK Docs.
DigitalInterrupt
API
Value
Get the current value of this interrupt.
Calculation of value differs between the "type"
of interrupt configured:
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt = await my_board.digital_interrupt_by_name(
name="my_example_digital_interrupt")
# Get the amount of times this DigitalInterrupt has been interrupted with a
# tick.
count = await interrupt.value()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Get the amount of times this DigitalInterrupt has been interrupted with a tick.
count, err := interrupt.Value(context.Background(), nil)
Parameters:
extra
(Optional[Dict[str, Any]]): Extra options to pass to the underlying RPC call.timeout
(Optional[float]): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (int): The RollingAverage of the time in nanoseconds between two successive low signals (pulse width) recorded by
Tick()
, computed over a window of size10
.
For more information, see the Python SDK Docs.
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt = await my_board.digital_interrupt_by_name(
name="my_example_digital_interrupt")
# Get the rolling average of the pulse width across each time the
# DigitalInterrupt is interrupted with a tick.
rolling_avg = await interrupt.value()
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (int64): The RollingAverage of the time in nanoseconds between two successive low signals (pulse width) recorded by
Tick()
, computed over a window of size10
. - (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Get the rolling average of the pulse width across each time the DigitalInterrupt is interrupted with a tick.
rolling_avg, err := interrupt.Value(context.Background(), nil)
Tick
Record an interrupt and notify any channels that have been added with AddCallback().
Tip
You should only need to integrate this method into your application code for testing purposes, as the handling of Tick()
should be automated once the interrupt is configured.
Calling this method is not yet fully implemented with the Viam Python SDK.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.high
(bool): Iftrue
, the state of the pin is set to high. Iffalse
, the state of the pin is set to low.now
(uint64): The time that has elapsed in nanoseconds since the last time the interrupt was ticked.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Record an interrupt and notify any interested callbacks.
err := interrupt.Tick(context.Background(), true, 12345)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.high
(bool): Iftrue
, the state of the pin is set to high. Iffalse
, the state of the pin is set to low.nanoseconds
(uint64): The time in nanoseconds between two successive low signals (pulse width).
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Record an interrupt and notify any interested callbacks.
err := interrupt.Tick(context.Background(), true, 12345)
AddCallback
Add a channel as a listener for when the state of the configured GPIO pin changes.
When Tick() is called, callbacks added to an interrupt will be sent the returned value high
.
Support Notice
This method is not available for digital interrupts configured with "type": "servo"
.
It is also not yet fully implemented with the Viam Python SDK.
Parameters:
callback
(chan Tick): The channel to add as a listener for when the state of the GPIO pin this interrupt is configured for changes between high and low.
Returns:
- None
For more information, see the Go SDK Docs.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Make the channel for Tick().
ch := make(chan Tick)
// Add the channel to "my_example_digital_interrupt" as a callback.
interrupt.AddCallback(ch)
Close
Safely shut down the resource and prevent further use.
Parameters:
- None
Returns:
- None
my_board = Board.from_robot(robot=robot, name="my_board")
# Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt = await my_board.digital_interrupt_by_name(
name="my_example_digital_interrupt")
# Close the interrupt.
await interrupt.close()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error) : An error, if one occurred.
myBoard, err := board.FromRobot(robot, "my_board")
// Get the DigitalInterrupt "my_example_digital_interrupt".
interrupt, ok := myBoard.DigitalInterruptByName("my_example_digital_interrupt")
// Close the interrupt.
err := interrupt.Close(ctx)
For more information, see the Go SDK Docs.
Troubleshooting
You can find additional assistance in the Troubleshooting section.
You can also ask questions in the Community Discord and we will be happy to help.
Next steps
Was this page helpful?
Glad to hear it! If you have any other feedback please let us know:
We're sorry about that. To help us improve, please tell us what we can do better:
Thank you!