Gantry Component
A robotic gantry is a mechanical system of linear actuators used to hold and position an end effector. A 3D printer is an example of a three-axis gantry where each linear actuator can move the print head along one axis. The linear rail design makes gantries a common and reliable system for simple positioning and placement tasks.
This component abstracts the hardware of a gantry to give you an easy interface for coordinated control of linear actuators, even many at once (multi-axis).
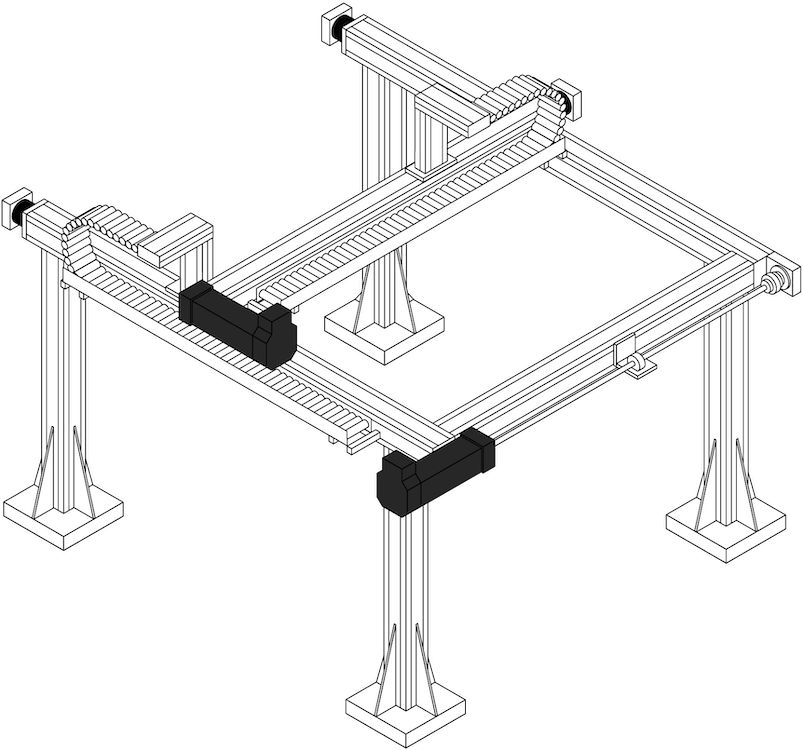
Gantry components can only be controlled in terms of linear motion (you cannot rotate them). Each gantry can only move in one axis within the limits of the length of the linear rail.
Most machines with a gantry need at least the following hardware:
- A board or controller component that can detect changes in voltage on GPIO pins
- A motor that can move linear rails
- Encoded motor: See DC motor with encoder and encoder component.
- Stepper motor: See Stepper motor. Requires setting limit switches in the config of the gantry, or setting offsets in the config of the stepper motor.
- Limit switches, to attach to the ends of the gantry’s axis
Related services
Supported models
To use your gantry component, check whether one of the following models supports it.
For configuration information, click on the model name:
Add support for other models
If none of the existing models fit your use case, you can create a modular resource to add support for it.
Support Notice
There is currently no support for this component in the micro-RDK.
Control your gantry with Viam’s client SDK libraries
To get started using Viam’s SDKs to connect to and control your machine, go to your machine’s page on the Viam app, navigate to the CONNECT tab’s Code sample page, select your preferred programming language, and copy the sample code generated.
API key and API key ID
By default, the sample code does not include your machine API key and API key ID. We strongly recommend that you add your API key and API key ID as an environment variable and import this variable into your development environment as needed.
To show your machine’s API key and API key ID in the sample code, toggle Include secret on the CONNECT tab’s Code sample page.
Caution
Do not share your API key or machine address publicly. Sharing this information could compromise your system security by allowing unauthorized access to your machine, or to the computer running your machine.
When executed, this sample code will create a connection to your machine as a client. Then control your machine programmatically by adding API method calls as shown in the following examples.
These examples assume you have a gantry called "my_gantry"
configured as a component of your machine.
If your gantry has a different name, change the name
in the code.
Be sure to import the gantry package for the SDK you are using:
from viam.components.gantry import Gantry
import (
"go.viam.com/rdk/components/gantry"
)
API
The gantry component supports the following methods:
Method Name | Description |
---|---|
GetPosition | Get the current positions of the axis of the gantry (mm). |
MoveToPosition | Move the axes of the gantry to the desired positions (mm) at the requested speeds (mm/sec). |
GetLengths | Get the lengths of the axes of the gantry (mm). |
Home | Run the homing sequence of the gantry to re-calibrate the axes with respect to the limit switches. |
GetGeometries | Get all the geometries associated with the gantry in its current configuration, in the frame of the gantry. |
IsMoving | Get if the gantry is currently moving. |
Stop | Stop all motion of the gantry. |
Reconfigure | Reconfigure this resource. |
DoCommand | Execute model-specific commands that are not otherwise defined by the component API. |
FromRobot | Get the resource from the provided robot with the given name. |
GetResourceName | Get the ResourceName for this gantry with the given name. |
Close | Safely shut down the resource and prevent further use. |
GetPosition
Get the current positions of the axis of the gantry (mm).
Parameters:
extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (List[float]): A list of the position of the axes of the gantry in millimeters.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
# Get the current positions of the axes of the gantry in millimeters.
positions = await my_gantry.get_position()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- ([]float64): A list of the position of the axes of the gantry in millimeters.
- (error): An error, if one occurred.
Example:
myGantry, err := gantry.FromRobot(machine, "my_gantry")
// Get the current positions of the axes of the gantry in millimeters.
position, err := myGantry.Position(context.Background(), nil)
For more information, see the Go SDK Docs.
MoveToPosition
Move the axes of the gantry to the desired positions (mm) at the requested speeds (mm/sec).
Parameters:
positions
(List[float]) (required): A list of positions for the axes of the gantry to move to, in millimeters.speeds
(List[float]) (required): A list of speeds in millimeters per second for the gantry to move at respective to each axis.extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- None.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
# Create a list of positions for the axes of the gantry to move to. Assume in
# this example that the gantry is multi-axis, with 3 axes.
examplePositions = [1, 2, 3]
exampleSpeeds = [3, 9, 12]
# Move the axes of the gantry to the positions specified.
await my_gantry.move_to_position(
positions=examplePositions, speeds=exampleSpeeds)
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.positionsMm
([]float64): A list of positions for the axes of the gantry to move to, in millimeters.speedsMmPerSec
([]float64): A list of speeds in millimeters per second for the gantry to move at respective to each axis.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
Example:
myGantry, err := gantry.FromRobot(machine, "my_gantry")
// Create a list of positions for the axes of the gantry to move to.
// Assume in this example that the gantry is multi-axis, with 3 axes.
examplePositions := []float64{1, 2, 3}
exampleSpeeds := []float64{3, 9, 12}
// Move the axes of the gantry to the positions specified.
myGantry.MoveToPosition(context.Background(), examplePositions, exampleSpeeds, nil)
For more information, see the Go SDK Docs.
GetLengths
Get the lengths of the axes of the gantry (mm).
Parameters:
extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (List[float]): A list of the lengths of the axes of the gantry in millimeters.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
# Get the lengths of the axes of the gantry in millimeters.
lengths_mm = await my_gantry.get_lengths()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- ([]float64): A list of the lengths of the axes of the gantry in millimeters.
- (error): An error, if one occurred.
Example:
myGantry, err := gantry.FromRobot(machine, "my_gantry")
// Get the lengths of the axes of the gantry in millimeters.
lengths_mm, err := myGantry.Lengths(context.Background(), nil)
For more information, see the Go SDK Docs.
Home
Run the homing sequence of the gantry to re-calibrate the axes with respect to the limit switches.
Parameters:
extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (bool): Whether the gantry has run the homing sequence successfully.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
await my_gantry.home()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (bool): Whether the gantry has run the homing sequence successfully.
- (error): An error, if one occurred.
Example:
myGantry, err := gantry.FromRobot(machine, "my_gantry")
myGantry.Home(context.Background(), nil)
For more information, see the Go SDK Docs.
Parameters:
Returns:
Example:
var homed = await myGantry.home();
For more information, see the Flutter SDK Docs.
GetGeometries
Get all the geometries associated with the gantry in its current configuration, in the frame of the gantry. The motion and navigation services use the relative position of inherent geometries to configured geometries representing obstacles for collision detection and obstacle avoidance while motion planning.
Parameters:
extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (List[viam.proto.common.Geometry]): The geometries associated with the Component.
Example:
geometries = await component.get_geometries()
if geometries:
# Get the center of the first geometry
print(f"Pose of the first geometry's centerpoint: {geometries[0].center}")
For more information, see the Python SDK Docs.
IsMoving
Get if the gantry is currently moving.
Parameters:
timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (bool): Whether the gantry is moving.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
# Stop all motion of the gantry. It is assumed that the
# gantry stops immediately.
await my_gantry.stop()
# Print if the gantry is currently moving.
print(my_gantry.is_moving())
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
Example:
// This example shows using IsMoving with an arm component.
myArm, err := arm.FromRobot(machine, "my_arm")
// Stop all motion of the arm. It is assumed that the arm stops immediately.
myArm.Stop(context.Background(), nil)
// Log if the arm is currently moving.
is_moving, err := myArm.IsMoving(context.Background())
logger.Info(is_moving)
For more information, see the Go SDK Docs.
Parameters:
- None.
Returns:
Example:
var moving = await myGantry.isMoving();
For more information, see the Flutter SDK Docs.
Stop
Stop all motion of the gantry.
Parameters:
extra
(Mapping[str, Any]) (optional): Extra options to pass to the underlying RPC call.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- None.
Example:
my_gantry = Gantry.from_robot(robot=robot, name="my_gantry")
# Stop all motion of the gantry. It is assumed that the gantry stops
# immediately.
await my_gantry.stop()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.extra
(map[string]interface{}): Extra options to pass to the underlying RPC call.
Returns:
- (error): An error, if one occurred.
Example:
// This example shows using Stop with an arm component.
myArm, err := arm.FromRobot(machine, "my_arm")
// Stop all motion of the arm. It is assumed that the arm stops immediately.
err = myArm.Stop(context.Background(), nil)
For more information, see the Go SDK Docs.
Parameters:
Returns:
- Future<void>
Example:
await myGantry.stop();
For more information, see the Flutter SDK Docs.
Reconfigure
Reconfigure this resource. Reconfigure must reconfigure the resource atomically and in place.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.deps
(Dependencies): The resource dependencies.conf
(Config): The resource configuration.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs.
DoCommand
Execute model-specific commands that are not otherwise defined by the component API.
For built-in models, model-specific commands are covered with each model’s documentation.
If you are implementing your own gantry and add features that have no built-in API method, you can access them with DoCommand
.
Parameters:
command
(Mapping[str, ValueTypes]) (required): The command to execute.timeout
(float) (optional): An option to set how long to wait (in seconds) before calling a time-out and closing the underlying RPC call.
Returns:
- (Mapping[str, viam.utils.ValueTypes]): Result of the executed command.
Raises:
- (NotImplementedError): Raised if the Resource does not support arbitrary commands.
Example:
command = {"cmd": "test", "data1": 500}
result = component.do(command)
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.cmd
(map[string]interface{}): The command to execute.
Returns:
- (map[string]interface{}): The command response.
- (error): An error, if one occurred.
Example:
// This example shows using DoCommand with an arm component.
myArm, err := arm.FromRobot(machine, "my_arm")
command := map[string]interface{}{"cmd": "test", "data1": 500}
result, err := myArm.DoCommand(context.Background(), command)
For more information, see the Go SDK Docs.
FromRobot
Get the resource from the provided robot with the given name.
Parameters:
robot
RobotClient (required)name
String (required)
Returns:
For more information, see the Flutter SDK Docs.
GetResourceName
Get the ResourceName
for this gantry with the given name.
Parameters:
name
(str) (required): The name of the Resource.
Returns:
- (viam.proto.common.ResourceName): The ResourceName of this Resource.
Example:
# Can be used with any resource, using an arm as an example
my_arm_name = my_arm.get_resource_name("my_arm")
For more information, see the Python SDK Docs.
Close
Safely shut down the resource and prevent further use.
Parameters:
- None.
Returns:
- None.
Example:
await component.close()
For more information, see the Python SDK Docs.
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error): An error, if one occurred.
Example:
// This example shows using Close with an arm component.
myArm, err := arm.FromRobot(machine, "my_arm")
err = myArm.Close(ctx)
For more information, see the Go SDK Docs.
Troubleshooting
You can find additional assistance in the Troubleshooting section.
You can also ask questions in the Community Discord and we will be happy to help.
Was this page helpful?
Glad to hear it! If you have any other feedback please let us know:
We're sorry about that. To help us improve, please tell us what we can do better:
Thank you!