Configure a TMC5072-Controlled Stepper Motor
The TMC5072
model supports stepper motors controlled by the TMC5072 chip.
Whereas a basic low-level stepper driver supported by the gpiostepper
model sends power to a stepper motor based on PWM signals from GPIO pins, the TMC5072 chip uses SPI bus to communicate with the board, does some processing on the chip itself, and provides convenient features including StallGuard2TM.
Navigate to the CONFIGURE tab of your machine’s page in the Viam app.
Click the + icon next to your machine part in the left-hand menu and select Component.
Select the motor
type, then select the TMC5072
model.
Enter a name or use the suggested name for your motor and click Create.
Edit and fill in the attributes as applicable.
{
"components": [
{
"name": "<your-board-name>",
"model": "<your-board-model>",
"type": "board",
"namespace": "rdk",
"attributes": {},
"depends_on": [],
},
{
"name": "<your-motor-name>",
"model": "TMC5072",
"type": "motor",
"namespace": "rdk",
"attributes": {
"spi_bus": "<your-spi-bus-index>",
"chip_select": "<pin-number>",
"index": "<your-terminal-index>",
"pins": {
"en_low": "<int>",
},
"ticks_per_rotation": <int>,
"max_acceleration_rpm_per_sec": <float>,
"sg_thresh": <int>,
"home_rpm": <float>,
"cal_factor": <float>,
"run_current": <int>,
"hold_current": <int>,
"hold_delay": <int>
},
"depends_on": []
}
]
}
{
"components": [
{
"name": "my-tmc-motor",
"model": "TMC5072",
"type": "motor",
"namespace": "rdk",
"attributes": {
"chip_select": "0",
"index": 1,
"pins": {
"en_low": "17"
},
"max_acceleration": 10000,
"max_rpm": 450,
"spi_bus": "0",
"ticks_per_rotation": 200
}
}
]
}
The following attributes are available for TMC5072
motors:
Name | Type | Required? | Description |
---|---|---|---|
spi_bus | string | Required | The index of the SPI bus over which the TMC chip communicates with the board. |
chip_select | string | Required | The chip select number (CSN) that the TMC5072 is wired to. For Raspberry Pis, use "0" if the CSN is wired to pin number 24 (GPIO 8) on the Pi, or use "1" if you wire the CSN to pin 26. The board sets this high or low to let the TMC chip know whether to listen for commands over SPI. |
pins | object | Required | A structure that holds the pin number you are using for "en_low" , the enable pin for the driver chip. |
index | int | Required | The index of the part of the chip the motor is wired to. Either 1 or 2 , depending on whether the motor is wired to the “MOTOR1” terminals or the “MOTOR2” terminals, respectively. |
ticks_per_rotation | int | Required | Number of full steps in a rotation. 200 (equivalent to 1.8 degrees per step) is very common. If your data sheet specifies this in terms of degrees per step, divide 360 by that number to get ticks per rotation. |
max_acceleration_rpm_per_sec | float | Optional | Set a limit on maximum acceleration in revolutions per minute per second. |
sg_thresh | int | Optional | Stallguard threshold; sets sensitivity of virtual endstop detection when homing. |
home_rpm | float | Optional | Speed in revolutions per minute that the motor will turn when executing a Home() command (through DoCommand()). |
cal_factor | float | Optional | Calibration factor for velocity and acceleration. Compensates for clock source drift when doing time-based calculations. |
run_current | int | Optional | Set current when motor is turning, from 1-32 as a percentage of rsense voltage. Defaults to 15 if omitted or set to 0. |
hold_current | int | Optional | Set current when motor is holding a position, from 1-32 as a percentage of rsense voltage. Defaults to 8 if omitted or set to 0. |
hold_delay | int | Optional | How long to hold full power at a set position before ramping down to hold_current . 0=instant powerdown, 1-15=delay * 2^18 clocks, 6 is the default. |
Refer to your motor and motor driver data sheets for specifics.
Extended API
The TMC5072
model supports additional methods that are not part of the standard Viam motor API (passed through DoCommand()):
Home
Home the motor using TMC’s StallGuardTM (a builtin feature of this controller).
Parameters:
- None
Raises:
- (error): An error, if one occurred.
For more information on do_command
, see the Python SDK Docs.
my_motor = Motor.from_robot(robot=robot, name='my_motor')
# Home the motor
home_dict = {
"command": "home"
}
await my_motor.do_command(home_dict)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK docs on Home() and on DoCommand().
// Home the motor
resp, err := myMotorComponent.DoCommand(ctx, map[string]interface{}{"command": "home"})
Jog
Move the motor indefinitely at the specified RPM.
Parameters:
rpm
(float64): The revolutions per minute at which the motor will turn indefinitely.
Raises:
- (error): An error, if one occurred.
For more information on do_command
, see the Python SDK Docs.
my_motor = Motor.from_robot(robot=robot, name='my_motor')
# Run the motor indefinitely at 70 rpm
jog_dict = {
"command": "jog",
"rpm": 70
}
await my_motor.do_command(jog_dict)
Parameters:
ctx
(Context): A Context carries a deadline, a cancellation signal, and other values across API boundaries.rpm
(float64): The revolutions per minute at which the motor will turn indefinitely.
Returns:
- (error): An error, if one occurred.
For more information, see the Go SDK Docs on Jog
and on DoCommand
.
// Run the motor indefinitely at 70 rpm
resp, err := myMotorComponent.DoCommand(ctx, map[string]interface{}{"command": "jog", "rpm": 70})
Test the motor
Once your motor is configured and connected, open the motor’s TEST panel on the CONFIGURE or CONTROL tabs. Use the buttons to try turning your motor forwards or backwards at different power levels and check whether it moves as expected.
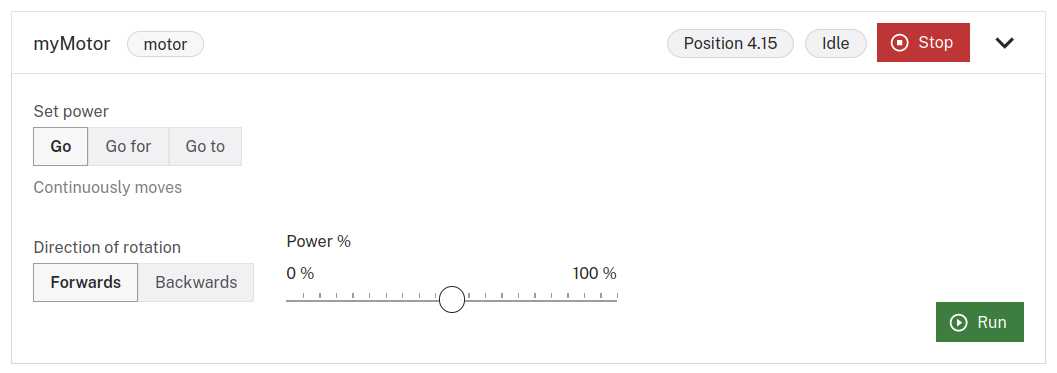
If the motor does not appear on the TEST panel, or if you notice unexpected behavior, check your machine’s LOGS tab for errors, and review the configuration.
Troubleshooting
If your motor is not working as expected, follow these steps:
- Check your machine logs on the LOGS tab to check for errors.
- Review this motor model’s documentation to ensure you have configured all required attributes.
- Check that all wires are securely attached to the correct pins.
- Click on the TEST panel on the CONFIGURE or CONTROL tab and test if you can use the motor there.
If none of these steps work, reach out to us on the Community Discord and we will be happy to help.
Next steps
For more configuration and usage info, see:
Have questions, or want to meet other people working on robots? Join our Community Discord.
If you notice any issues with the documentation, feel free to file an issue or edit this file.
Was this page helpful?
Glad to hear it! If you have any other feedback please let us know:
We're sorry about that. To help us improve, please tell us what we can do better:
Thank you!